KDGpu v.0.1.0 is released a Vulkan wrapper to make modern graphics easier
We’re pleased to announce we’ve added a new library, KDGpu, to the arsenal of tools we invent to make our lives easier – and then share with you on KDAB’s GitHub.
Who is this for?
If you want to become more productive with Vulkan or learn the concepts of modern explicit graphics APIs, then KDGpu is the library for you!
KDGpu is a thin wrapper around Vulkan whose purpose it is to make modern graphics APIs more accessible and easier to learn. It cuts through the verbose syntax, makes managing object lifetimes much simpler and allows you to get your project working without having to be bogged down in intricacies involved in tasks such as synchronization or memory handling. The sensible option defaults in KDGpu mean that you can focus on solving the problem at hand. Furthermore, KDGpu exposes almost all of the power of raw Vulkan and is very well suited to teaching modern graphics APIs and their concepts.
KDGpu enables you to make examples like this easily and with great readability:
What got KDAB started on this?
As you are likely aware, KDAB offers training in 3D. So far, we have used OpenGL as the vehicle for these training courses and for years it has performed very well, both for the courses and the industry.
However, with the modern explicit graphics APIs that have come along (Vulkan, Metal, D3D12 and WebGPU), OpenGL has been left to languish a bit. On MacOS, Apple has frozen OpenGL at version 4.1 which is annoying as 4.2 brought all sorts of cool things to the party.
Replacing OpenGL in our training courses with Vulkan wasn’t really an option due to the extreme complexity in this, the most verbose API available. We needed something like Vulkan but not Vulkan 🙂
Say hello to KDGpu!
What does KDGpu Provide?
Although we began work on KDGpu as a tool to aid teaching, it has grown beyond that into a library that is suitable for use in production projects and we are using it as such ourselves. We will keep adding to the library as we find new features that we need but we will keep KDGpu itself as lightweight as possible. It is also possible that in the future we may add other backend beyond Vulkan.
For now though, what exactly is in KDGpu the repository?
- The KDGpu library. A collection of classes and options structs that make it easy, concise and clear to work with Vulkan.
- An example framework called KDGpuExample that provides integration with KDGui to make it easy to experiment by providing a cross-platform windowing and event loop implementation.
- A set of illustrative examples showing how to use KDGpu for common rendering tasks from the typical hello_triangle through to rendering involving multiple rendering and compute passes.
KDGpu is independent of any particular windowing system. You can use it purely with platform native APIs or you can checkout the included KDGpuExample library which makes it trivial to use KDGpu with KDGui::Window and friends. Check out the handy KDBindings repository too for some more syntactic sugar.
The following images were all created with examples written with KDGpu. We will publish some more in depth blogs on these and other examples in the future to show just how easy it is to reason about graphics issues without getting lost in hundreds of lines of code.
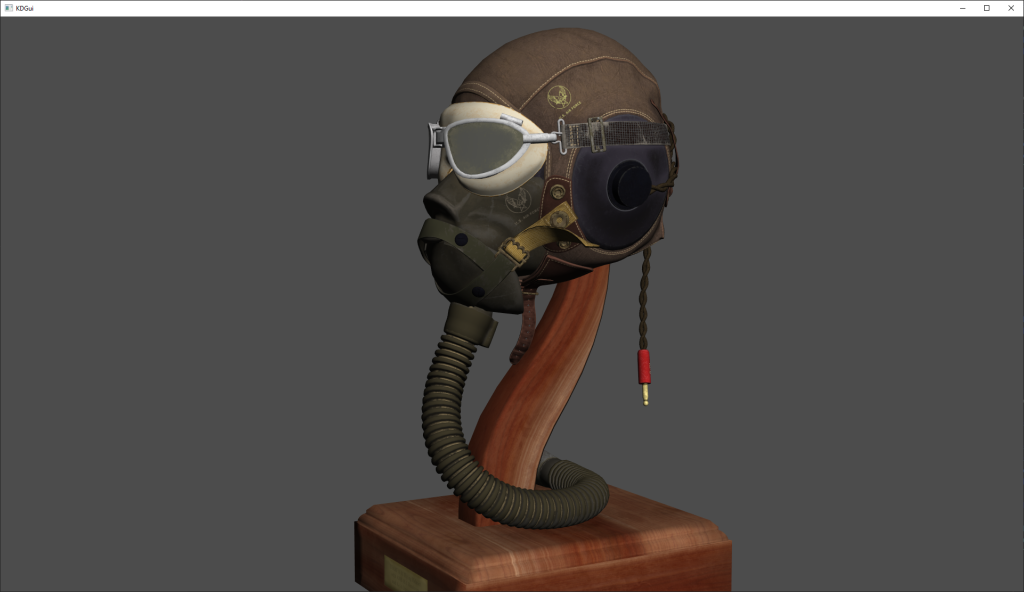
Using KDGpu to render a textured glTF 2 model. KDGpu makes it easy to manage resources for shaders. The glTF parsing was performed using the excellent tinygltf library.

Instanced rendering of the glTF 2 buggy model. Each sub-mesh is drawn using instancing to reduce the number of draw calls and binding operations.
For now, you can get started looking at the examples in the repository and looking at the documentation.
The KDGpuExample helper library is a great help to those starting out. It takes care of creating a device; performing frame-to-frame synchronisation (options for loops with and without the use of vkDeviceWaitIdle); managing the depth buffer; easily enabling of MSAA etc. When you want to take the leap and manage these things yourself or integrate KDGpu into your own engine, then it is very easy to do so.
Show me some code!
We won’t go into all of the details in this announcement blog, but it would be remiss of us not to give you a taster of what KDGpu code looks like in practise.
The core of a graphics application is its render function which gets called each and every frame to record the command buffers for the GPU to process. In KDGpu, the process of recording a command buffer to bind a graphics pipeline, set some state and actually draw something would look like this:
void HelloTriangle::render()
{
// m_device is a KDGpu::Device
auto commandRecorder = m_device.createCommandRecorder();
// m_opaquePassOptions is a KDGpu::RenderPassCommandRecorderOptions struct that we use to specify the render pass setup
m_opaquePassOptions.colorAttachments[0].view = m_swapchainViews.at(m_currentSwapchainImageIndex);
auto opaquePass = commandRecorder.beginRenderPass(m_opaquePassOptions);
// Get ready to draw
opaquePass.setPipeline(m_pipeline);
opaquePass.setVertexBuffer(0, m_buffer);
opaquePass.setIndexBuffer(m_indexBuffer);
opaquePass.setBindGroup(0, m_transformBindGroup);
// Record the draw command
const DrawIndexedCommand drawCmd = { .indexCount = 3 };
opaquePass.drawIndexed(drawCmd);
// End the render pass and finish the command recording
opaquePass.end();
m_commandBuffer = commandRecorder.finish();
// Submit command buffer
...
}
In the above code, we are referring to a few other objects of types such as KDGpu::Buffer (m_buffer and m_indexBuffer), KDGpu::BindGroup (m_transformBindGroup) and KDGpu::GraphicsPipeline (m_pipeline). The functions that consume these objects (and many others) are actually passing around handles to the objects rather than the objects themselves.
The objects themselves are owning, move-only, strong references to the underlying Vulkan objects whereas the templated Handle type is a weak-reference that is very cheap to pass around. The handles are just generational indices into some object pools managed by KDGpu.
To keep a GPU resource alive, simply keep the corresponding C++ object alive. To pass it to a consuming function, each class has a convenience conversion to handle operator implemented. This really simplifies resource management with natural C++ semantics.
Just having local named objects makes the explicit graphics APIs much easier to reason about than OpenGL’s massive global state machine approach. Using KDGpu to go even further in terms of reducing code verbosity and using it’s sane defaults approach makes graphics programming a delight and accessible to mere mortals.
Of course, rendering is not very exciting without some GPU-based resources such as textures and buffers. KDGpu makes creating such resources very easy and intuitive.
We begin with a quick look at the KDGpu::BufferOptions struct:
struct BufferOptions {
DeviceSize size;
BufferUsageFlags usage;
MemoryUsage memoryUsage;
SharingMode sharingMode{ SharingMode::Exclusive };
std::vector queueTypeIndices{};
};
Of note is the sensible default value for the sharing mode member. The option is there if we need to set it but in the common case we can just run with the default value. The code to create a buffer object to hold some index data is then pretty much as you would hope:
std::array<uint32_t, 3> indexData = { 0, 1, 2 };
const DeviceSize dataByteSize = indexData.size() * sizeof(uint32_t);
const BufferOptions bufferOptions = {
.size = dataByteSize,
.usage = BufferUsageFlagBits::IndexBufferBit | BufferUsageFlagBits::TransferDstBit,
.memoryUsage = MemoryUsage::GpuOnly
};
m_indexBuffer = m_device.createBuffer(bufferOptions);
The memory usage flags indicate that we wish the buffer to be resident in GPU memory and the usage flags indicate that we will use it as a source of index data for some geometry and that the buffer should be capable of being the target of a copy operation (we need to get the data in there somehow).
This pattern of using options structs and initializing them with C++20 designated initializers permeates through the API. It makes it easily discoverable, extensible and trivial to queue up for deferred invocations.
Uploading data to the above buffer is just as simple:
const BufferUploadOptions uploadOptions = {
.destinationBuffer = m_indexBuffer,
.dstStages = PipelineStageFlagBit::VertexAttributeInputBit,
.dstMask = AccessFlagBit::IndexReadBit,
.data = indexData.data(),
.byteSize = dataByteSize
};
m_queue.uploadBufferData(uploadOptions);
Here we are using the uploadBufferData function that is a member function on KDGpu::Queue to perform a synchronous upload of the data. If you instead wish to perform an asynchronous upload of the data to keep the CPU doing useful work, then the KDGpuExample library has helpers for this too. There is some interplay with the concept of a frame which the KDGpu::Queue on its own does not know about. Feel free to take a look at the code for the details, it is all very simple and clearly written.
Find out more and get up and running with KDGpu
In this blog post we have introduced KDAB’s new library, KDGpu, seen some of the advantages it brings, and had a cursory look at a flavour of the API in use.
If this has whetted your appetite for GPU programming, look out for our follow-up posts which will deal with glTF rendering and more in much more detail. We think that you will like KDGpu. We have certainly had fun writing it and then using it on projects. For now, please give KDGpu a try.
If you like this article and want to read similar material, consider subscribing via our RSS feed.
Subscribe to KDAB TV for similar informative short video content.
KDAB provides market leading software consulting and development services and training in Qt, C++ and 3D/OpenGL. Contact us.